1.6 Inheritance
There are two fundamental mechanisms for building new classes from existing ones: inheritance and aggregation (p. 16). It makes sense to inherit from an existing class Vehicle to define a class Car, since a car is a vehicle. The class Vehicle has several parts; therefore, it makes sense to define a composite object of the class Vehicle that has constituent objects of such classes as Engine, Axle, and GearBox, which make up a vehicle.
Inheritance is illustrated here by an example that implements a point in three-dimensional space—that is, a 3D point represented by (x, y, z)-coordinates. We can derive the 3D point from the Point2D class. This 3D point will have all the properties and behaviors of the Point2D class, along with the additional third dimension. This relationship is shown in Figure 1.5 and implemented in Example 1.3. The class Point3D is called the subclass, and the class Point2D is called the superclass. The Point2D class is a generalization for points, whereas the class Point3D is a specialization of points that have three coordinates.
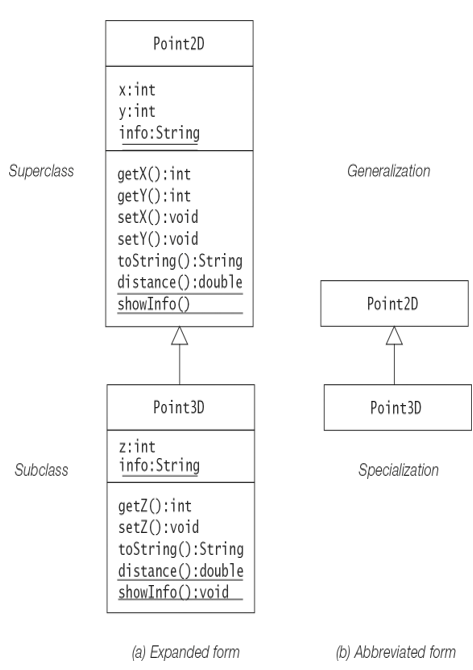
Figure 1.5 Class Diagram Depicting Inheritance Relationship
In Java, deriving a new class from an existing class requires the use of the extends clause in the subclass declaration. A subclass can extend only one superclass. The subclass Point3D extends the Point2D class, shown at (1).
public class Point3D extends Point2D { // (1) Uses extends clause
// …
}
The Point3D class only declares the z-coordinate at (3), as every object of the subclass will have the x and y fields that are specified in its superclass Point2D. Note that these fields are declared private in the superclass Point2D, but they are accessible to a Point3D object indirectly through the public get and set methods for the x- and the y-coordinates in the superclass Point2D. These methods are inherited by the Point3D class.
The constructor of the Point3D class at (4) takes three arguments corresponding to the x-, y-, and z-coordinates. The call to super() at (5) results in the constructor of the superclass Point2D being called to initialize the x- and y-coordinates.
It addition, the Point3D class declares methods at (6) to get and set the z-coordinate. It provides its own version of the toString() method to format a point that has three coordinates.
Since calculating the distance is also different in three-dimensional space from that in a two-dimensional plane, the Point3D class provides its own distance() static method at (7). As its objects represent 3D points, it declares its own static field info and provides its own static method showInfo() to print this information.
Example 1.3 Defining a Subclass
// File: Point2D.java
public class Point2D {
// Same as in Example 1.2.
}
// File: Point3D.java
public class Point3D extends Point2D { // (1) Uses extends clause
// Static variable: (2)
private static String info = “A 3D point represented by (x,y,z)-coordinates.”;
// Instance variable: (3)
private int z;
// Constructor: (4)
public Point3D(int xCoord, int yCoord, int zCoord) {
super(xCoord, yCoord); // (5)
z = zCoord;
}
// Instance methods: (6)
public int getZ() { return z; }
public void setZ(int zCoord) { z = zCoord; }
@Override
public String toString() {
return “(” + getX() + “,” + getY() + “,” + z + “)”; // Format: (x,y,z)
}
// Static methods: (7)
public static double distance(Point3D p1, Point3D p2) {
int xDiff = p1.getX() – p2.getX();
int yDiff = p1.getY() – p2.getY();
int zDiff = p1.getZ() – p2.getZ();
return Math.sqrt(xDiff*xDiff + yDiff*yDiff + zDiff*zDiff);
}
public static void showInfo() { System.out.println(info); }
}
Objects of the Point3D class will respond just like objects of the Point2D class, but they also have the additional functionality defined in the subclass. References of the class Point3D are used in the code below. The comments indicate in which class a method is invoked. Note that the subclass reference can invoke the inherited get and set methods in the superclass.
Point3D p3A = new Point3D(10, 20, 30);
System.out.println(p3A.toString()); // (10,20,30) (Point3D)
System.out.println(“x: ” + p3A.getX()); // x: 10 (Point2D)
System.out.println(“y: ” + p3A.getY()); // y: 20 (Point2D)
System.out.println(“z: ” + p3A.getZ()); // z: 30 (Point3D)
p3A.setX(-10); p3A.setY(-20); p3A.setZ(-30);
System.out.println(p3A.toString()); // (-10,-20,-30) (Point3D)
Point3D p3B = new Point3D(30, 20, 10);
System.out.println(p3B.toString()); // (30,20,10) (Point3D)
System.out.println(Point3D.distance(p3A, p3B)); // 69.2820323027551 (Point3D)
Point3D.showInfo(); // A 3D point represented by (x,y,z)-coordinates. (Point3D)