1.2 Classes
One of the fundamental ways in which we handle complexity is by using abstractions. An abstraction denotes the essential properties and behaviors of an object that differentiate it from other objects. The essence of OOP is modeling abstractions, using classes and objects. The hardest part of this endeavor is coming up with the right abstractions.
A class denotes a category of objects, and acts as a blueprint for creating objects. A class models an abstraction by defining the properties and behaviors of the objects representing the abstraction. An object exhibits the properties and behaviors defined by its class. The properties of an object of a class are also called attributes, and are defined by fields in Java. A field in a class is a variable that can store a value that represents a particular property of an object. The behaviors of an object of a class are also known as operations, and are defined using methods in Java. Fields and methods in a class declaration are collectively called members.
An important distinction is made between the contract and the implementation that a class provides for its objects. The contract defines which services are provided, and the implementation defines how these services are provided by the class. Clients (i.e., other objects) need only know the contract of an object, and not its implementation, to avail themselves of the object’s services.
As an example, we will implement a class that models the abstraction of a point as (x, y)-coordinates in a two-dimensional plane. The class Point2D will use two int fields x and y to store the coordinates. Using simplified Unified Modeling Language (UML) notation, the class Point2D is graphically depicted in Figure 1.1, which models the abstraction. Both fields, with their type and method names and their return value type, are shown in Figure 1.1a.
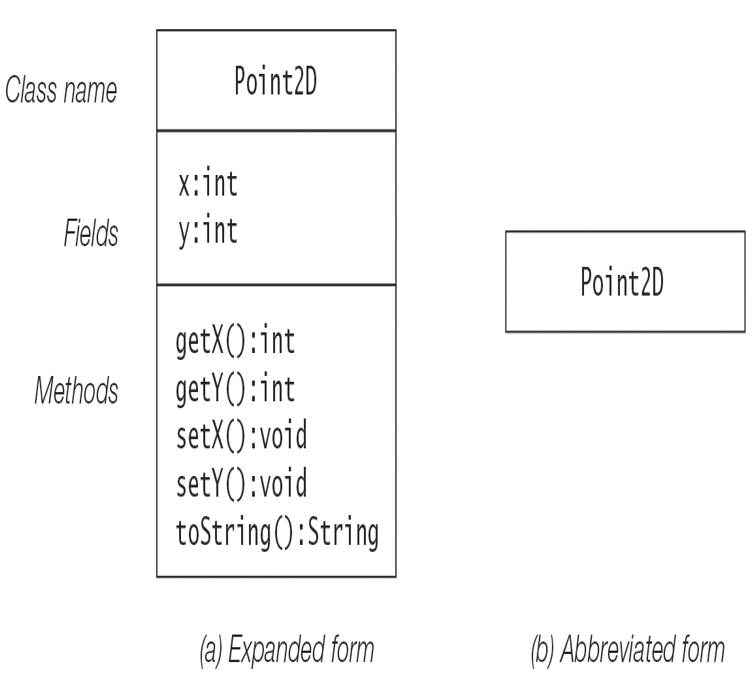
Figure 1.1 UML Notation for Classes