1.7 Aggregation
An association defines a static relationship between objects of two classes. One such association, called aggregation (also known as composition), expresses how an object uses other objects. Java supports aggregation of objects by reference, since objects cannot contain other objects explicitly. The aggregate object usually has fields that denote its constituent objects. By default, Java uses aggregation when fields denoting objects are declared in a class declaration. Typically, an aggregate object delegates its tasks to its constituent objects.
We illustrate aggregation by implementing a finite-length straight line that has two end points in a two-dimensional plane. We would like to use the class Point2D to implement such a line. A class Line could be implemented by having fields for two Point2D objects that would represent the end points of a line. This aggregate relationship is depicted in Figure 1.6, which shows that a Line object has two Point2D objects, indicated by the diamond notation. The complete declaration of the Line class is shown in Example 1.4. The two fields endPoint1 and endPoint2 declared at (1) represent the two end points. In particular, note the length() method at (2) which delegates the computation of the length to the Point2D.distance() method.
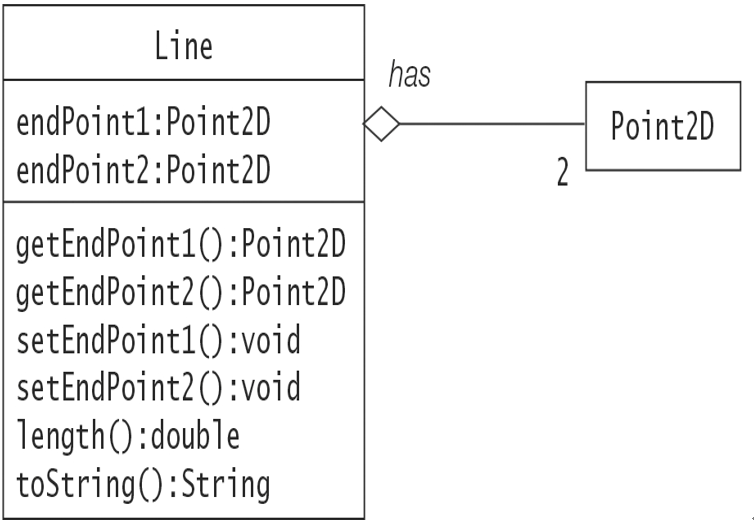
Figure 1.6 Class Diagram Depicting Aggregation
The following code shows how a Line object can be manipulated:
Line line1 = new Line(new Point2D(5,6), new Point2D(7,8));
System.out.println(line1.toString()); // Line[(5,6),(7,8)]
line1.setEndPoint1(new Point2D(11, 12));
line1.setEndPoint2(new Point2D(13, 14));
System.out.println(line1.toString()); // Line[(11,12),(13,14)]
System.out.println(“Length: ” + line1.length()); // Length: 2.8284271247461903
// File: Point2D.java
public class Point2D {
// Same as in Example 1.2.
}
// File: Line.java
public class Line {
// Instance variables: (1)
private Point2D endPoint1;
private Point2D endPoint2;
// Constructor:
public Line(Point2D p1, Point2D p2) {
endPoint1 = p1;
endPoint2 = p2;
}
// Methods:
public Point2D getEndPoint1() { return endPoint1; }
public Point2D getEndPoint2() { return endPoint2; }
public void setEndPoint1(Point2D p1) { endPoint1 = p1; }
public void setEndPoint2(Point2D p2) { endPoint2 = p2; }
public double length() { // (2)
return Point2D.distance(endPoint1, endPoint2);
}
public String toString() {
return “Line[” + endPoint1 + “,” + endPoint2 + “]”;
}
}